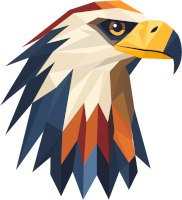
Business Intelligence as a Service
Try PlusClouds Eaglet service and find high quality B2B hot leads and opportunites with AI support.
What is Axios?
In its simplest form, it is a JavaScript library that allows us to easily perform HTTP operations in client-side applications.
Axios Installation
We can install it via npm, yarn, and CDN.
Installation with Npm
$ npm install axios
Installation with Yarn
$ yarn add axios
Installation with CDN
A Small Example with the Axios.get() Method
Let's create a small API example with Axios's get() method
import axios from 'axios' // we import axios to use it after loading
export default {
data(){
return{
users:null, // I created an empty variable named users
}},
methods:{
function (){
axios.get('https://jsonplaceholder.typicode.com/users').then((response) => {
// we fetch the api and use the then() method for further operations
this.users = response.data; // we accessed the data object within the returned response and assigned it to the variable named users
console.log(this.users); // now we logged the returned results to the console
})
}
}
}
Console Output
Let's Visualize the Result in the Example
Let's create a small table with the help of Bootstrap and place the data coming from the API into this table
And the Result…